Generate Key Number Javascript Map Array
by Alex Permyakov
- Generate Key Number Javascript Map Array Of Objects
- Generate Key Number Javascript Map Array In Excel
- Javascript Array Number Of Elements
- Javascript For Each
- Javascript Generate Array Of Numbers
- Javascript Key Value Array
When you read about Array.reduce and how cool it is, the first and sometimes the only example you find is the sum of numbers. This is not our definition of ‘useful’. ?
Moreover, I’ve never seen it in a real codebase. But, what I’ve seen a lot is 7–8 line for-loop statements for solving a regular task where Array.reduce could do it in one line.
Recently I rewrote a few modules using these great functions. It surprised me how simplified the codebase became. So, below is a list of goodies.
Therefore, Array(N) is insufficient; Array(N).map(Number.call, Number) would result in an uninitialized array of length N. Compatibility Since this technique relies on behaviour of Function.prototype.apply specified in ECMAScript 5, it will not work in pre-ECMAScript 5 browsers such.
If you have a good example of using a map or reduce method — post it in the comments section. ?
Let’s get started!
1. Remove duplicates from an array of numbers/strings
Well, this is the only one not about map/reduce/filter, but it’s so compact that it was hard not to put it in the list. Plus we’ll use it in a few examples too.
- Iterable objects (objects such as Map and Set). Array.from has an optional parameter mapFn, which allows you to execute a map function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map(mapFn, thisArg), except that it does not create an intermediate array.
- Sep 14, 2017 In this article we would be discussing Map object provided by ES6.Map is a collection of elements where each element is stored as a Key, value pair. Map object can hold both objects and primitive values as either key or value. When we iterate over the map object it returns the key,value pair in the same order as inserted.
- The Array.of method creates a new Array instance from a variable number of arguments, regardless of number or type of the arguments. The difference between Array.of and the Array constructor is in the handling of integer arguments: Array.of(7) creates an array with a single element, 7, whereas Array(7) creates an empty array with a length property of 7 (Note: this implies an array of 7.
- Arrays are a special type of objects. The typeof operator in JavaScript returns 'object' for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its 'elements'. In this example, person0 returns John.
2. A simple search (case-sensitive)
The filter() method creates a new array with all elements that pass the test implemented by the provided function.
3. A simple search (case-insensitive)
4. Check if any of the users have admin rights
The some() method tests whether at least one element in the array passes the test implemented by the provided function.
5. Flattening an array of arrays
The result of the first iteration is equal to : […[], …[1, 2, 3]] means it transforms to [1, 2, 3] — this value we provide as an ‘acc’ on the second iteration and so on.
We can slightly improve this code by omitting an empty array[]
as the second argument for reduce(). Then the first value of the nested will be used as the initial acc value. Thanks to Vladimir Efanov.
Note that using the spread operator inside a reduce is not great for performance. This example is a case when measuring performance makes sense for your use-case. ☝️
Thanks to Paweł Wolak, here is a shorter way without Array.reduce:
Also Array.flat is coming, but it’s still an experimental feature.
6. Create an object that contains the frequency of the specified key
Generate Key Number Javascript Map Array Of Objects
Let’s group and count the ‘age’ property for each item in the array:
Thanks to sai krishna for suggesting this one!
7. Indexing an array of objects (lookup table)
Instead of processing the whole array for finding a user by id, we can construct an object where the user’s id represents a key (with constant searching time).
It’s useful when you have to access your data by id like uTable[85].name
a lot.
8. Extract the unique values for the given key of each item in the array
Let’s create a list of existing users’ groups. The map() method creates a new array with the results of calling a provided function on every element in the calling array.
9. Object key-value map reversal
This one-liner looks quite tricky. We use the comma operator here, and it means we return the last value in parenthesis — acc
. Let’s rewrite this example in a more production-ready and performant way:
Here we don’t use spread operator — it creates a new array on each reduce() call, which leads to a big performance penalty: O(n²). Instead the old good push() method.

10. Create an array of Fahrenheit values from an array of Celsius values
Think of it as processing each element with a given formula ?
11. Encode an object into a query string
12. Print a table of users as a readable string only with specified keys
Sometimes you want to print your array of objects with selected keys as a string, but you realize that JSON.stringify is not that great ?
JSON.stringify can make the string output more readable, but not as a table:
13. Find and replace a key-value pair in an array of objects
Let’s say we want to change John’s age. If you know the index, you can write this line: users[1].age = 29
. However, let’s take a look at another way of doing it:
Here instead of changing the single item in our array, we create a new one with only one element different. Now we can compare our arrays just by reference like updatedUsers users
which is super quick! React.js uses this approach to speed up the reconciliation process. Here is an explanation.
14. Union (A ∪ B) of arrays
Less code than importing and calling the lodash method union.
15. Intersection (A ∩ B) of arrays
The last one!
As an exercise try to implement difference (A B) of the arrays. Hint: use an exclamation mark.
Thanks to Asmor and incarnatethegreat for their comments about #9.
That’s it!
If you have any questions or feedback, let me know in the comments down below or ping me on Twitter.
If this was useful, please click the clap ? button down below a few times to show your support! ⬇⬇ ??
Here are more articles I’ve written:
Production ready Node.js REST APIs Setup using TypeScript, PostgreSQL and Redis.
A month ago I was given a task to build a simple Search API. All It had to do is to grab some data from 3rd party…
Thanks for reading ❤️
Now we’ve learned about the following complex data structures:
- Objects for storing keyed collections.
- Arrays for storing ordered collections.
But that’s not enough for real life. That’s why Map
and Set
also exist.
Map
Map is a collection of keyed data items, just like an Object
. But the main difference is that Map
allows keys of any type.
Methods and properties are:
new Map()
– creates the map.map.set(key, value)
– stores the value by the key.map.get(key)
– returns the value by the key,undefined
ifkey
doesn’t exist in map.map.has(key)
– returnstrue
if thekey
exists,false
otherwise.map.delete(key)
– removes the value by the key.map.clear()
– removes everything from the map.map.size
– returns the current element count.
For instance:
As we can see, unlike objects, keys are not converted to strings. Any type of key is possible.
Generate Key Number Javascript Map Array In Excel
Although map[key]
also works, e.g. we can set map[key] = 2
, this is treating map
as a plain JavaScript object, so it implies all corresponding limitations (no object keys and so on).
So we should use map
methods: set
, get
and so on.
Map can also use objects as keys.
For instance:
Using objects as keys is one of most notable and important Map
features. For string keys, Object
can be fine, but not for object keys.
Let’s try:
As visitsCountObj
is an object, it converts all keys, such as john
to strings, so we’ve got the string key '[object Object]'
. Definitely not what we want.
Javascript Array Number Of Elements
To test keys for equivalence, Map
uses the algorithm SameValueZero. It is roughly the same as strict equality , but the difference is that NaN
is considered equal to NaN
. So NaN
can be used as the key as well.
This algorithm can’t be changed or customized.
Every map.set
call returns the map itself, so we can “chain” the calls:
Iteration over Map
For looping over a map
, there are 3 methods:
map.keys()
– returns an iterable for keys,map.values()
– returns an iterable for values,map.entries()
– returns an iterable for entries[key, value]
, it’s used by default infor.of
.
For instance:
The iteration goes in the same order as the values were inserted. Map
preserves this order, unlike a regular Object
.
Besides that, Map
has a built-in forEach
method, similar to Array
:
Sep 03, 2012 I Am Alive v1.0 All No-DVD Reloaded i installed and crack this game but, keys W,A,S,D, Space not working. Only ctrl n mouse working. I Am Alive 2012 serial number, Crack Download. I Am Alive 2012 serial number is full of adventurous action in the post apocalyptic I am alive, the players are competed with provoking selects the choices with the role of a survivor on a journey he is find his wife and daughter in the journey who were lost by the Event that arriving at his hometown of haven ton, this happened after one year, so.
Object.entries: Map from Object
When a Map
is created, we can pass an array (or another iterable) with key/value pairs for initialization, like this:
If we have a plain object, and we’d like to create a Map
from it, then we can use built-in method Object.entries(obj) that returns an array of key/value pairs for an object exactly in that format.
So we can create a map from an object like this:
Here, Object.entries
returns the array of key/value pairs: [ ['name','John'], ['age', 30] ]
. That’s what Map
needs.
Object.fromEntries: Object from Map
We’ve just seen how to create Map
from a plain object with Object.entries(obj)
.
There’s Object.fromEntries
method that does the reverse: given an array of [key, value]
pairs, it creates an object from them:
We can use Object.fromEntries
to get an plain object from Map
.
Javascript For Each
E.g. we store the data in a Map
, but we need to pass it to a 3rd-party code that expects a plain object.
Here we go:
A call to map.entries()
returns an array of key/value pairs, exactly in the right format for Object.fromEntries
.
We could also make line (*)
shorter:
That’s the same, because Object.fromEntries
expects an iterable object as the argument. Not necessarily an array. And the standard iteration for map
returns same key/value pairs as map.entries()
. So we get a plain object with same key/values as the map
.
Set
A Set
is a special type collection – “set of values” (without keys), where each value may occur only once.
Its main methods are:
new Set(iterable)
– creates the set, and if aniterable
object is provided (usually an array), copies values from it into the set.set.add(value)
– adds a value, returns the set itself.set.delete(value)
– removes the value, returnstrue
ifvalue
existed at the moment of the call, otherwisefalse
.set.has(value)
– returnstrue
if the value exists in the set, otherwisefalse
.set.clear()
– removes everything from the set.set.size
– is the elements count.
The main feature is that repeated calls of set.add(value)
with the same value don’t do anything. That’s the reason why each value appears in a Set
only once.
For example, we have visitors coming, and we’d like to remember everyone. But repeated visits should not lead to duplicates. A visitor must be “counted” only once.
Set
is just the right thing for that:
The alternative to Set
could be an array of users, and the code to check for duplicates on every insertion using arr.find. But the performance would be much worse, because this method walks through the whole array checking every element. Set
is much better optimized internally for uniqueness checks.
Iteration over Set
Javascript Generate Array Of Numbers
We can loop over a set either with for.of
or using forEach
:
Note the funny thing. The callback function passed in forEach
has 3 arguments: a value
, then the same valuevalueAgain
, and then the target object. Indeed, the same value appears in the arguments twice.
That’s for compatibility with Map
where the callback passed forEach
has three arguments. Looks a bit strange, for sure. But may help to replace Map
with Set
in certain cases with ease, and vice versa.
The same methods Map
has for iterators are also supported:
set.keys()
– returns an iterable object for values,set.values()
– same asset.keys()
, for compatibility withMap
,set.entries()
– returns an iterable object for entries[value, value]
, exists for compatibility withMap
.
Summary
Javascript Key Value Array
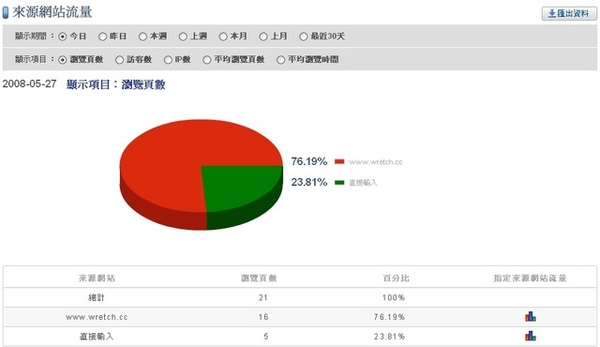
Map
– is a collection of keyed values.
Methods and properties:
new Map([iterable])
– creates the map, with optionaliterable
(e.g. array) of[key,value]
pairs for initialization.map.set(key, value)
– stores the value by the key.map.get(key)
– returns the value by the key,undefined
ifkey
doesn’t exist in map.map.has(key)
– returnstrue
if thekey
exists,false
otherwise.map.delete(key)
– removes the value by the key.map.clear()
– removes everything from the map.map.size
– returns the current element count.
The differences from a regular Object
:
- Any keys, objects can be keys.
- Additional convenient methods, the
size
property.
Set
– is a collection of unique values.
Methods and properties:
new Set([iterable])
– creates the set, with optionaliterable
(e.g. array) of values for initialization.set.add(value)
– adds a value (does nothing ifvalue
exists), returns the set itself.set.delete(value)
– removes the value, returnstrue
ifvalue
existed at the moment of the call, otherwisefalse
.set.has(value)
– returnstrue
if the value exists in the set, otherwisefalse
.set.clear()
– removes everything from the set.set.size
– is the elements count.
Iteration over Map
and Set
is always in the insertion order, so we can’t say that these collections are unordered, but we can’t reorder elements or directly get an element by its number.